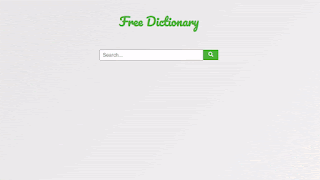
In this article, we will fabricate an exceptionally straightforward Dictionary application with the assistance of an API. This is an ideal undertaking for novices as it will show you how to bring data from an API and show it and a few essentials of how React really functions. Likewise, we will find out about how to utilize React symbols. How about we start.
Approach: Our application contains two areas, one for taking the client input and the other is for showing the information. At whatever point a client looks for a word, we store that contribution to a state and trigger an API call dependent on the looked through watchword boundary. After that when the API call is made, we just store that API reaction in another state variable, and afterward we at last presentation the data in like manner.
Prerequisites: The pre-requisites for this project are:
- React
- Functional Components
- React Hooks
- React Axios & API
- javascript ES6
Creating a React application and installing some npm packages:
Step 1: Create a react application by typing the following command in the terminal:
npx create-react-app dictionary-app
Step 2: Now, go to the project folder i.e dictionary-app by running the following command:
cd dictionary-app
Step 3: Let’s install some npm packages required for this project:
npm install axios
npm install react-icons --save
Project Structure: It should look like this:
Example: It is the lone segment of our application that contains all the rationale. We will utilize a free opensource API (no auth required) called
'Free Dictionary API' to bring every one of the necessary information. Our application contains two areas i.e a part for taking the client input and a hunt button, the other is for showing the information. Aside from showing the data that we got, we will likewise have a speaker button that allows clients to pay attention to the phonetics.
Presently record the accompanying code in the App.js document. Here, the App is our default segment where we have composed our code. Here, the filename is App.js and App.css
javascript
import { React, useState } from "react";
import Axios from "axios";
import "./App.css";
import { FaSearch } from "react-icons/fa";
import { FcSpeaker } from "react-icons/fc";
function App() {
// Setting up the initial states using react hook 'useState'
const [data, setData] = useState("");
const [searchWord, setSearchWord] = useState("");
// Function to fetch information on button
// click, and set the data accordingly
function getMeaning() {
Axios.get(
`https://api.dictionaryapi.dev/api/v2/entries/en_US/${searchWord}`
).then((response) => {
setData(response.data[0]);
});
}
// Function to play and listen the
// phonetics of the searched word
function playAudio() {
let audio = new Audio(data.phonetics[0].audio);
audio.play();
}
return (
<div className="App">
<h1>Free Dictionary</h1>
<div className="searchBox">
// Taking user input
<input
type="text"
placeholder="Search..."
onChange={(e) => {
setSearchWord(e.target.value);
}}
/>
<button
onClick={() => {
getMeaning();
}}
>
<FaSearch size="20px" />
</button>
</div>
{data && (
<div className="showResults">
<h2>
{data.word}{" "}
<button
onClick={() => {
playAudio();
}}
>
<FcSpeaker size="26px" />
</button>
</h2>
<h4>Parts of speech:</h4>
<p>{data.meanings[0].partOfSpeech}</p>
<h4>Definition:</h4>
<p>{data.meanings[0].definitions[0].definition}</p>
<h4>Example:</h4>
<p>{data.meanings[0].definitions[0].example}</p>
</div>
)}
</div>
);
}
export default App;
HTML:
@import url(
'https://fonts.googleapis.com/css2?family=Pacifico&display=swap');
@import url(
'https://fonts.googleapis.com/css2?family=Poppins:ital,wght@0,200;0,400;0,600;0,800;1,300&display=swap');
.App {
height: 100vh;
width: 100vw;
display: flex;
flex-direction: column;
align-items: center;
background-color: #f6f6f6;
background-image: linear-gradient(315deg, #f6f6f6 0%, #e9e9e9 74%);
font-family:'Poppins', sans-serif;
}
h1 {
text-align: center;
font-size: 3em;
font-family: 'Pacifico', cursive;
color: #4DB33D;
padding: 1.5em;
}
h2{
font-size: 30px;
text-decoration: underline;
padding-bottom: 20px;
}
h4{
color: #4DB33D;
}
input{
width: 400px;
height: 38px;
font-size: 20px;
padding-left: 10px;
}
.searchBox > button{
background-color: #4DB33D;
height: 38px;
width: 60px;
border: none;
color: white;
box-shadow: 0px 3px 2px #439e34;
cursor: pointer;
padding: 0;
}
.showResults{
width: 500px;
padding: 20px;
}
.showResults > h2 > button{
background: none;
border: none;
cursor: pointer;
}
Step to Run Application: Run the application using the following command from the root directory of the project:
npm start
Output: Now open your browser and go to http://localhost:3000/, you will see the following output:
#viastudy
0 Comments